Real-Time Collaboration in SaaS Applications Using SignalR in .NET
In real-time applications, data is given to subscribers as soon as it is published by the publisher or author. Real-time applications are utilized in a variety of industries, including banking (fraud detection, transaction management, trading), transportation (air traffic control systems), collaboration (Google Docs), and social networking (Facebook, Twitter, and Google+). We have developed real-time apps employing a variety of technologies, including HTTP long polling, Forever Frame, Server Sent Events, and WebSockets. All of these technologies have certain restrictions. Microsoft introduced SignalR, which is included in the ASP.NET frameworks. It uses existing transport protocols to enable real-time communication while addressing concerns with conventional real-time technology.
Introduction to SignalR
SignalR is a library that simplifies adding real-time web functionality to applications. It allows server-side code to push content to connected clients instantly. SignalR handles connection management automatically, and it scales out to handle large numbers of connections.
Key Features of SignalR
- Real-time Communication : Enables bi-directional communication between server and client.
- Automatic Reconnection: Automatically reconnects if the connection is dropped.
- Multiple Transport Options: Chooses the best transport method (WebSocket, Server-Sent Events, Long Polling) depending on the client’s capabilities.
Overview of transport options
Server-Sent Events (SSE) / HTTP Server Push
In the server push technique, the connection is sustained until all data is delivered to the client. Unidirectional communication protocol where the server can push updates to the client over an HTTP connection. The push is always initiated by the client. Server sends data to the clients periodically when there are updates. Unlike WebSocket, SSE only supports server-to-client communication.
Long Polling / HTTP Client Pull
In the client pull technique, a new HTTP connection is opened every time a document is requested. In a predefined period (Time to Refresh) the client calls the web server to receive updated data regardless of the presence (or the lack of) of any updates. Amongst the versions of HTTP client pull could be found
- HTTP polling (request response) – client makes a request and waits for the server to respond . If there is an update it is sent to the client. If there isn’t one the server sends an empty response
- HTTP long polling (request wait response) – Client creates a connection to the server, and it stays opened for a period of time. Within this time slot the client can receive data from the server. The connection is closed right after the server sends its response
WebSocket
WebSockets is a full-duplex communication protocol that establishes a persistent connection between a client and a server. This allows data to be sent in both directions simultaneously without repeatedly opening and closing connections.
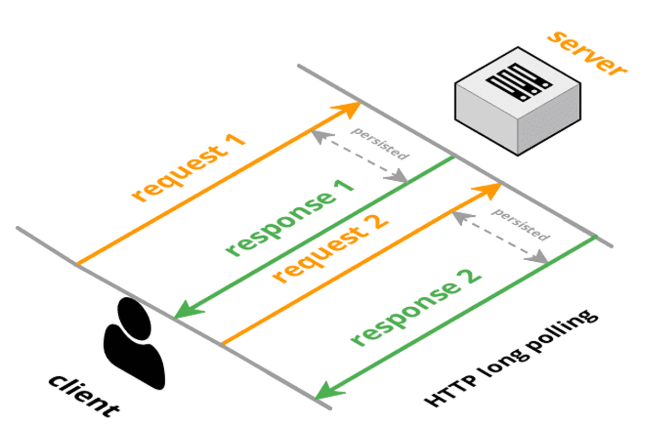
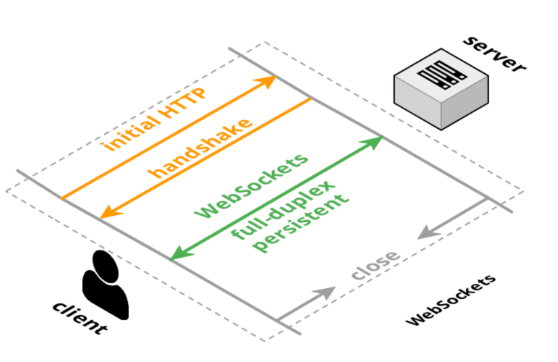
SignalR Transport Mechanism
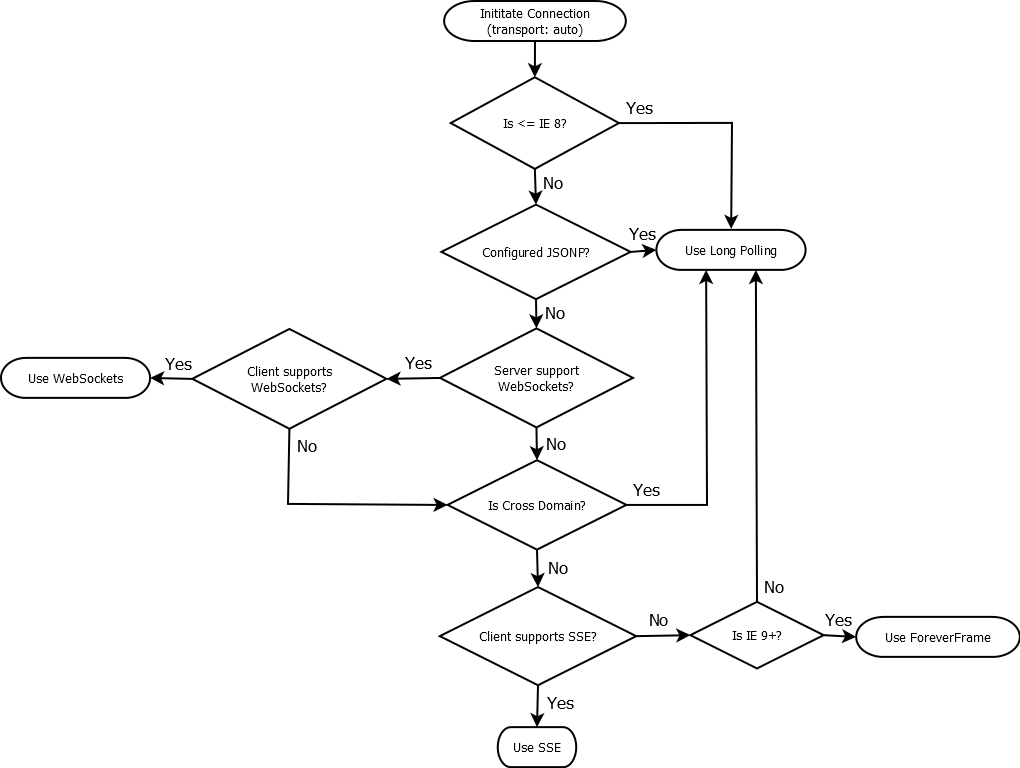
Use cases of real time apps
With technology gaining importance in all aspects of our existence around the world, real-time technology on mobile and web is one the fastest emerging areas of computing. Realtime web will be the foundation of the next web (say Web 3.0). There are various use cases of real-time apps.
Banking and Financial Services (BFS)
In the BFS industry, real-time technologies are used in various areas mainly in capital markets where several push-based messaging patterns are used. For instance, clients subscribe to services that publish stock prices, broadcast messages & real time charts etc.
E-Commerce
Real-time technologies allow retailers to watch as customers shop on their sites and accordingly offer discounts and customer support to convert web visits into sales. Showing the related products or pushing online hot deals to all connected customers is the real-time apps features for e-commerce segment
Online Games
Multiplayer online games depend on low latency communication between players and this is one of the key use cases for real-time technology.
Collaboration
Large organizations are increasingly emphasizing on collaboration between teams as this impacts productivity of team members positively and can cut cost significantly. There are various tools in the market that are used for collaboration such as on-line meetings, web-based trainings, in-browser IDE and so on
Chat
Chat is the de-facto example of real-time technology as it requires bi-directional real-time communication between chat users. With the evolution of real-time technologies, there are various opportunities to modernize chat applications.
Real-Time Progress Tracking Using SignalR
This example demonstrates real-time progress tracking for a ZIP file upload process using SignalR. It provides a mechanism for the server to send updates about the upload progress to connected clients in real time. This is particularly useful in applications where users need immediate feedback during long-running operations, such as uploading or processing files.
Step 1: Frontend SignalR Setup (Angular)
1. Install SignalR Client
Install the SignalR client library for Angular
npm install @microsoft/signalr
2. Create a SignalR Service
Create a service (signalr.service.ts
) to manage the SignalR connection.
import { Injectable } from '@angular/core';
import * as signalR from '@microsoft/signalr';
import { BehaviorSubject } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class SignalRService {
private hubConnection: signalR.HubConnection;
private progressSubject = new BehaviorSubject<number>(0);
public progress$ = this.progressSubject.asObservable();
startConnection() {
this.hubConnection = new signalR.HubConnectionBuilder()
.withUrl('https://your-backend-url/signalr-hub') // Update with your backend hub URL
.build();
this.hubConnection
.start()
.then(() => console.log('SignalR connection started'))
.catch((err) => console.log('Error while starting SignalR connection:', err));
this.hubConnection.on('ReceiveProgress', (progress: number) => {
this.progressSubject.next(progress);
});
}
stopConnection() {
if (this.hubConnection) {
this.hubConnection.stop();
}
}
}
3. Integrate SignalR in the Component
Use the SignalR service in your Angular component
OpenPopUpMessageBox() {
this.messageTitle = 'Photo Selection Status';
this.showPopUpMessage = true;
this.selectedAllImagesCheckbox = false;
this.isDeleteSelectedImages = false;
this.uploadProgress = 0;
this.signalRService.startConnection();
this.signalRService.progress$.subscribe((progress) => {
this.uploadProgress = progress;
});
}
Step 2: Backend SignalR Setup (ASP.NET Core)
1. Install SignalR Package
Install SignalR for your ASP.NET Core backend
dotnet add package Microsoft.AspNetCore.SignalR
2. Create a SignalR Hub
The SignalR Hub is the central point of communication for all connected clients. Here’s how we define our hub
using Microsoft.AspNetCore.SignalR;
public class ProgressHub : Hub
{
// You can define additional methods if needed
}
3. Register the Hub in Startup
Add SignalR to your ASP.NET Core app in Startup.cs
or Program.cs
.
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ProgressHub>("/signalr-hub");
});
}
Step 3: Upload Progress Simulation (Backend)
1. Send Progress Updates from the Backend
Use the SignalR hub context to send real-time progress updates.
private readonly IHubContext<ProgressHub> _hubContext;
public YourController(IHubContext<ProgressHub> hubContext)
{
_hubContext = hubContext;
}
[HttpPost("UploadZippedPhotos")]
public async Task<IActionResult> UploadZippedPhotos([FromBody] ZippedImages item)
{
// Simulating chunk upload progress
for (int i = 0; i < item.TotalChunks; i++)
{
// Simulate processing each chunk
await Task.Delay(100); // Simulate some delay
var progress = (i + 1) * 100 / item.TotalChunks;
// Send progress to SignalR clients
await _hubContext.Clients.All.SendAsync("ReceiveProgress", progress);
}
return Ok(new { Status = "Completed" });
}
Step 4: User Interface Updates
1. Progress Bar in Angular
Update the UI to display the upload progress.
<div *ngIf="showPopUpMessage">
<h2>{{ messageTitle }}</h2>
<div *ngIf="uploadProgress > 0">
<p>Upload Progress: {{ uploadProgress }}%</p>
<div class="progress-bar">
<div class="progress" [style.width]="uploadProgress + '%'"></div>
</div>
</div>
</div>
Step 5: Test the Application
Test the backend by ensuring the SignalR hub is functional and sends accurate progress updates, while the chunk upload logic correctly handles file sizes, types, and errors. On the frontend, verify that real-time progress updates are displayed seamlessly, and test with large files to confirm smooth progress bar behavior.
Why SignalR is a good fit for real-time collaboration in SaaS
SignalR is a robust library in the .NET ecosystem designed to enable real-time web functionality, making it an excellent fit for SaaS applications requiring collaborative features. Here’s why:
- Real-Time Communication Capabilities
- Multiple Transport Options for Compatibility
- Simplified API for Developers
- Scalability and Performance for SaaS
- Integration with the .NET Ecosystem
SignalR’s ability to deliver real-time functionality with minimal developer effort, combined with its scalability, security, and cross-platform compatibility, makes it a natural choice for SaaS applications. Whether it’s collaborative document editing, live notifications, or real-time analytics, SignalR equips developers to build high-performance, reliable, and engaging real-time features tailored to the needs of modern SaaS users.
Conclusion
Real-time collaboration has become a cornerstone of modern SaaS applications, enhancing user engagement and productivity. SignalR, with its seamless integration into the .NET ecosystem, offers a robust and flexible solution for implementing real-time features. By leveraging SignalR’s intelligent transport selection, scalable architecture, and simple API, developers can create dynamic and interactive applications that cater to diverse business needs.
While challenges like scalability and network reliability may arise, the benefits of real-time communication far outweigh these hurdles. From chat applications to collaborative tools and live dashboards, SignalR enables developers to deliver exceptional user experiences. By following best practices and utilizing the tools provided by SignalR, you can bring your SaaS applications to the forefront of real-time innovation.
Whether you’re building a small chat feature or a complex collaborative environment, SignalR empowers your applications to connect users in meaningful and efficient ways. Now is the time to harness the power of real-time communication and make your SaaS offerings truly stand out.